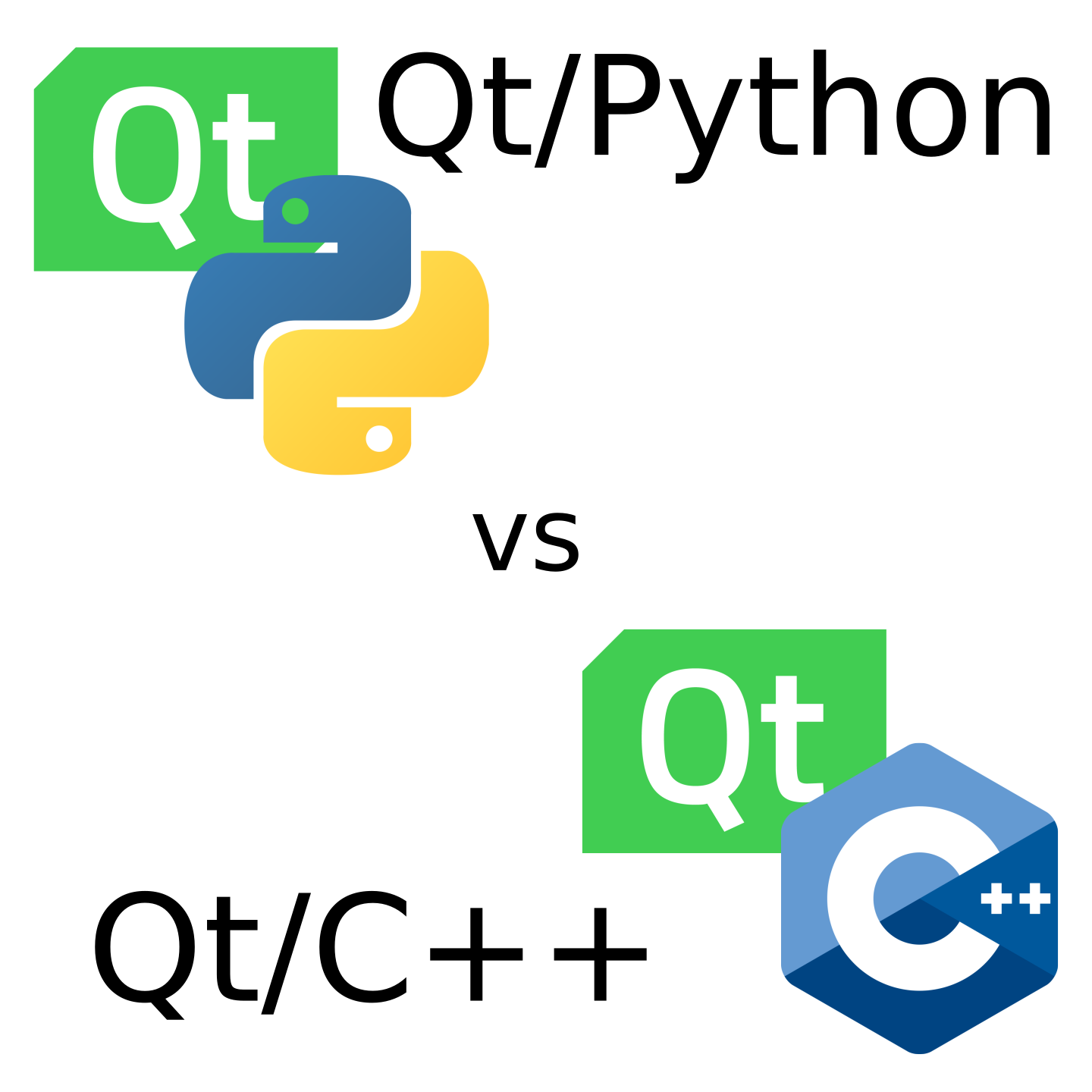
In this article, you will learn the differences between choosing Python or C++ as programming language when working with the Qt framework.
In many aspects, the comparison between Qt/C++ vs. Qt/Python boils down to comparing C++ with Python. However, in some points, the particular differences between the Python bindings for Qt and the native Qt/C++ implementation are a major deciding factor regardless of your programming language preference. Therefore, I encourage you to read this article, even if you have a strong preference for one or the other programming language.
From C++ to Python
It was September 2012 at university when I first was introduced to the programming language Python. At first, Python felt completely alien to me, coming from C++ and C as main programming languages.
It took me two more years to actively use Python for something bigger than a few shell script replacements when I started the Machinekit open source motion control software project with a few fellow open source developers.
Although I used Python in a few Gtk GUI projects, I haven't seen Python as a good alternative for C++ in combination with Qt until December of 2017. One of the biggest reasons for me was the portability of my code for different platforms, including Windows, Linux, OS X and, Android.
However, in December 2017 I started to work on a Linux-only project with PyQt. The work on these projects opened my eyes. I literally could do the same things I did with Qt/C++ with Python but in roughly one-third of the time.
Since then, I use the Python Qt combination in more and more projects and, I don't regret my choice.
PyQt vs. PySide / Qt for Python
Confusing for most people when starting with Qt and, Python is the fact that there a two different Python bindings for Qt, PyQt and PySide2 also known as Qt for Python.
In short, Qt for Python is the officially supported binding and in most cases the way to go. For more details, you can read my article about this topic.
Lines of Code
Lines of code are not the best measure for code quality or developer efficiency. However, it is proven that shorter code is more maintainable than longer code.
I'm not talking about clever one-liners, which are often hard to read, but the overall lines of code it takes to implement a particular feature.
When comparing different programming languages, the same features takes roughly ten times the amount of LOCs to implement in a low-level language such as C vs. a high-level programming language such as Python.
When comparing system languages, such as C++ to high-level language such as Python, the factor goes down to roughly three, which is still a lot in terms of maintainability and also development effort.
Where does this difference in the amount of code needed to implement a project come from? Partly from the differences in syntax, Python does not use braces, but mostly due to the extensive standard library and high-level language features. As people say, Python comes batteries included.
When comparing Qt/C++ to Python, we need to keep in mind that Qt strongly complements the features of the C++ standard library. Therefore, in comparison to raw C++, especially before C++11, we end up with a comparison factor roughly around 2.5
Development Speed
One of the biggest advantages of Python compared to C++ is the development speed of projects with this language.
But why is it so much faster to develop a project with Python compared to C++? Here are the top 5 reasons I discovered:
- Python is a scripting language, no need to recompile the code.
- Python has a simpler syntax, no braces.
- Python comes with batteries included, also known as the extensive standard library.
- Python uses dynamic-typing, no need to define data types when not necessary.
- Python has a package manager and a huge collection of open source libraries.
Overall, these factors multiply the development velocity of a Python Qt project compared to a C++ Qt project manifold. Since developer times is valuable, this fact should definitely be considered when choosing the programming language for a project.
Popularity
The Python programming language has become very popular in the last few years due to the rise of data science and machine learning.
Python is ranked as the third most popular programming language on GitHub. In comparison, C++ ranks as the fifth most popular programming language.
You may ask why it is essential that the programming language you choose for a project is widespread. The answer is simple, with popularity comes to a bigger community, more libraries, more developers and higher discovery rate of issues in the programming language and its tools.
From a business standpoint, the popularity of a programming language and tools is also very important. It doesn't matter if you are a small or big company or if you prefer working with contractors or employing developers, it is significantly harder to find someone already pre-qualified to work on your project if the programming language or framework you choose is very niche.
Of course, a sound engineer can quickly learn a new framework or even a new programming language. In my opinion, it's more important that the developer is good in solving a certain problem, such as creating HMIs, rather than working with specific tools.
However, the overall starting phase of a project is significantly shorter when people working on the project are already familiar with the tools and languages. This is especially crucial for small projects.
Portability and Deployment
One primary reason for choosing Qt over let's say the platforms native GUI framework is the possibility to run one application on multiple platforms with a single code base.
C++ and Python are both platform independent programming languages. However, platform support and deployment significantly vary between the two.
Almost all platforms I know support C++ as a programming language, especially if they are capable of running a Qt-based user interface. On the contrary, Python is not supported on many embedded systems and only poorly supported by the dominant mobile OS.
If you are going for mobile and desktop support or your target is an embedded device, Qt for Python is no valid choice at the moment. PyQt claims to support Android and iOS, but other developers and I failed to reproduce the claims.
Also important to mention is, that Qt/C++ provides consistent tooling for all platforms. This includes deployment tool, remote debugging and the Qt Creator IDE, which is my opinion an excellent choice for Qt/C++ developers.
Another recent development is Qt for WebAssembly. In short, Qt for WASM enables you to deploy your Qt/C++ applications in WebAssembly format, which means they run inside a web browser on all WebAssembly supported OS.
In my opinion, WebAssembly is a major game-changer in web frontend and desktop UI world. It combines the advantages of web applications, including continuous and straightforward deployment, with the benefits of the system UI development world.
Python is currently not well supported by WebAssembly, but things might change in the future. If you want to try out web development with Qt soon, choose Qt/C++.
Quality Assurance
Python and C++ are two inherently different programming languages. How to apply Quality Assurance (QA) measures to a project strongly depends on the programming language; the differences between the Python bindings of Qt and Qt/C++ are less relevant.
A huge difference between Python and C++ is that Python uses so-called duck-typing and C++ uses static typing. Also in Python, types are dynamically assigned and changed at runtime, which can lead to various runtime errors on the boundary to external systems and when input types aren't checked correctly.
In C++, many type errors and mistakes can be caught when compiling the application. Similar static analysis tools are available for Python, but many conditions can only be evaluated at runtime. Therefore, it's even more critical to test Python code thoroughly.
Another essential difference between C++ and Python is how memory management works. Qt/C++ uses a parent-child relationship in its object to free up memory. Python, on the other hand, has a garbage collector. In C++ it's straightforward to build applications with memory leaks when not being careful. Compared to C++ and even Qt's memory management, Python is a lot easier to use, at the cost flexibility.
Moreover, it is impossible at the moment to certify Python code for safety-relevant applications such as required in the automotive or aerospace industries.
Testing
When it comes to testing, Python starts to shine again. Pythons duck-typing makes testing-related tasks such as writing mocks for classes and modules very easy and straightforward.
Moreover, Python as a scripting language does not need to be recompiled for running unit tests, which makes test-driven development (TDD) much faster and more fun to work with.
I also would like to highlight the Python testing framework Pytest at this point. Pytest makes writing tests as comfortable as possible. A simple unit test is no more than a Python function inside a Python file next to the source code of the Python module to test. Compared to that, writing a test in C++ is usually a lot more work.
When it comes to testing, keeping things simple is critical. Developers are lazy (that's usually a good thing) so simpler test code increases the likelihood of tests to be written during development and not in the last minute before completing a project.
Performance
When comparing Python and C++ people quickly begin to talk about performance. In almost all Benchmarks, Python programs are significantly slower than C++ applications.
Most benchmarks compare the performance of computing mathematical and algorithmic problems or I/O intensive tasks. C++ is significantly more suitable for these types of applications. With the right library usage, for example numpy
for mathematical applications, the performance of Python can be improved, but still, will never reach the performance of a C++ application.
But what's most important for us is, how the does Qt for Python perform compared to Qt/C++. For this purpose, benchmarking the computational and I/O performance of the programming language and interpreter does not help us.
Especially front-end and HMI applications are idle most of the time. The only thing that matters when creating an HMI application is that the UI interactions are as fast possibly perceivable by a human. We don't want to have a UI that feels sluggish and slow, and we don't need a UI that can be operated by super-humans.
Features and Issues
- PyQt / Qt for Python less used -> less tested
- Binding bugs on top Qt/C++ bugs
- some showstoppers, but infrequently
- most just annoying and undocumented (see my blog post)
Another vital factor to consider when choosing Qt/Python over Qt/C++ is the number of open issues and missing features. In short, since the Python bindings for Qt wrap the Qt/C++ bindings, you will get the problems of the Python binding on top of Qt's issues.
Moreover, it's worth mentioning that both Qt/Python bindings have combined fewer users than Qt/C++ and, therefore, bugs and issues are later or never discovered. For example, I found at least three problems when working on a bigger project with Qt and Python, which were related entirely to the Python bindings and not the Qt framework itself.
However, I found only a few real showstoppers, most of the bugs and issues I found where just undocumented behavior which could be worked around.
Development Environment
Choosing a development environment for Qt/Python is very different from selecting a development environment for Qt/C++. For Qt/C++, Qt Creator is an excellent choice, but we also have other options such as MS Visual Studio with the Qt plugin.
However, when searching for an IDE that supports both, Qt and Python very well, we quickly run out of options. Either we have good Qt/QML support or we have good Python support.
The Qt Company is working on better support for Python in Qt Creator, but in the meantime, I recommend to use a good Python IDE, and either use Qt Creator for the Qt Quick/Widget designer or the QML support most IDEs provide.
I personally use CLion as IDE, since it supports both C++ and Python (it integrates PyCharm) as well QML. The Qt/QML support is not perfect, but I can live with that, since I use live-coding.
Summary and Conclusion
The following graphic summarizes all the differences between Qt/C++ and Qt/Python.
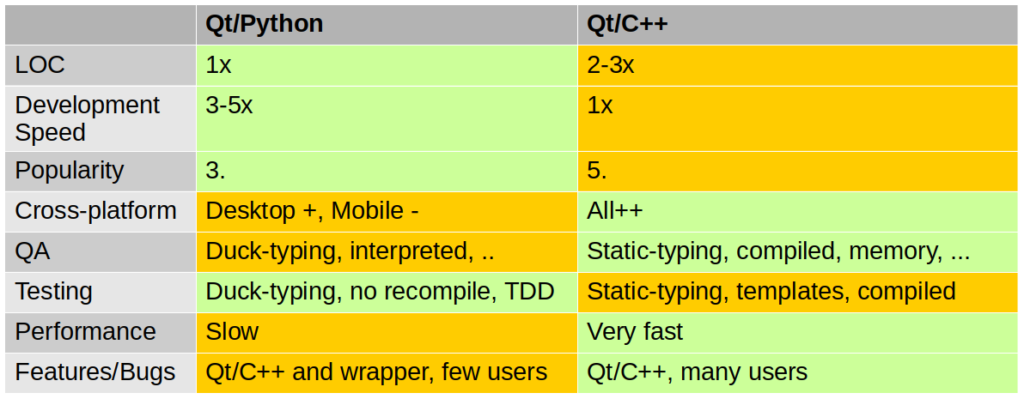
Qt/Python and Qt/C++ comparison chart
Having Python as an option for writing the business logic of your Qt application is tremendous and drastically speeds up development. It certainly opens up new possibilities due to the enormous ecosystem of the Python programming language and community.
On the other hand, the Qt for Python bindings are less tested and contain more issues compared to the C++ equivalents. Moreover, Python is not a suitable programming language for safety-relevant applications. Little support for mobile and embedded applications might be another issue relevant for your use case.
In short, I can recommend trying out Qt/Python for your next application if you are not working in a safety critical environment and you are not interested in mobile support at the moment. With Qt, you are always free to use C++ for the performance critical tasks if necessary. I'm very sure your business and project will benefit from the Pythons development efficiency boost.
Your
Machine Koder